0
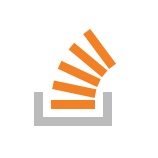
I've got a simulation I'm playing with, in which I have a large (1024x1024 or bigger) euclidean space. Each square of this space can hold various data, of fixed but not totally trivial size. (Below is an example square; while it is very subject to change, I don't expect it to grow more by a fact...