1
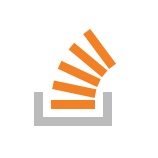
Instead of lerping from 0.0 to 1.0 between two colors, you need to scale it times 3. 0..1 would be Red to Yellow, 1..2 would be Yellow to Green and 2..3 would be Green to Blue. You can reuse this function to create a transition for each block.
I haven't written everything down, but maybe this is ...