43
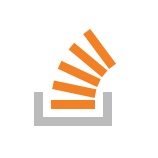
While looking at the code for Dear Imgui, I found the following code (edited for relevance):
struct ImVec2
{
float x, y;
float& operator[] (size_t idx) { return (&x)[idx]; }
};
It's pretty clear that this works in practice, but from the perspective of the C++ standard, is this code legal...