1
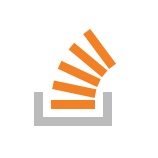
fgets() in fgets(name,50,stdin); reads AND includes the '\n' as part of the buffer it fills (name in this example).
To remove the '\n' simply overwrite it with a '\0'. To remove the newline from the end of any string, you can use:
name[strcspn (name, "\n")] = 0;
strcspn (name, "\n") returns th...