-2
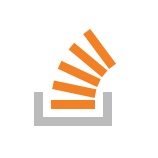
&str[0] is safe to use -- so long as you do not assume it points to a null-terminated string.
Since C++11 the requirements include (section [string.accessors]):
str.data() and str.c_str() point to a null-terminated string.
&str[i] == str.data() + i , for 0 <= i <= str.size()
note that this imp...