0
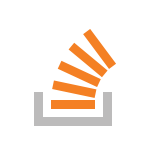
I want to export the image in the picture box to a location in the HDD, this image is set to the picturebox from an image type column in MSSQL database table.
I have already written the following code to acheive this task. However I get a generic GDI+ error by the try catch code.
This code e...