0
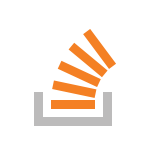
I was asked to sort 50,000 random integers (from 0 to 1000) with heap sort, bubble sort, and selection sort, to see which method is most efficient. My bubble and selection sort works fine, but I noticed my heap sort did not sort correctly. I re-used my heap sort from another program and it worked...