0
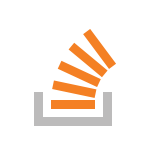
So basically we usually define cross entropy like this:
dim = 5
logits = tf.random_normal([5,3],dtype=tf.float32)
labels = tf.cast(tf.one_hot(10,5),tf.int32)
cost_entropy = tf.nn.sparse_softmax_cross_entropy_with_logits(logits=logits,labels=labels)
with tf.Session() as sess:
a,b=sess....