0
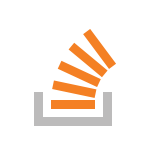
I was looking to implement an subject-observer pattern where the subject provides its self to the observers when notifying.
public class Subject<T extends Subject> {
/** suporting stuff for subject */
private List<Observer<T>> observers = new ArrayList<>();
protected void doNotif...