0
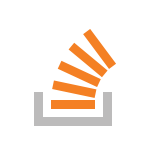
Well, something like this works, I'm sure there are better ways, but at the moment I'm not up to thinking really:
// Declare Scanner Object to read our file
Scanner in = new Scanner(new File(stringRepresentingLocationOfYourFileHere));
// create Map that will contain keys in sorted o...