-1
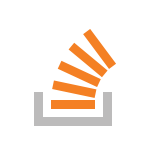
I am currently thinking about ways to store memory contiguously (due to cache) in cases where said memory can be added and deleted at run-time.
In other words, I'm trying to make a vector of vectors with contiguous memory.
I have thought of various ways to do this, one of them being to instead s...