-3
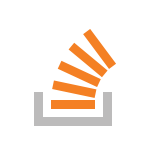
In languages like Common Lisp you can use closures to hide global variables, as in
(let ((my-global-variable '(foo bar'))))
(defun f (x) (append my-global-variable x)))
One possible way to do this in Python is
def helper_function():
my_global_variable = ['foo', 'bar']
def f(x):
...