1
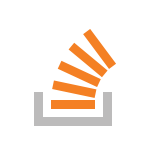
There's already quite a lot going on, so I might have missed a few things.
One thing seems odd: both player are using the same Projectile pointer when they shoot!
Player 1 shoots: a first projectile is heap allocate and you keep its address in p. So far so good.
Then Player 2 shoots. You creat...