1
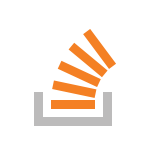
I suggest that you start by commenting out Square and Triangle so that you can focus on your Rectangle (or whichever shape you prefer).
Your GeometricShape needs an abstract property called "area" see https://msdn.microsoft.com/en-us/library/yd3z1377.aspx
Your Rectangle constructor should not ...