1
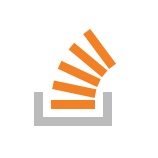
I suggest that you delete the following global variables
int P1_CUR_AMT = 200;
int P2_CUR_AMT = 200;
int P1_CUR_POS = 0;
int P2_CUR_POS = 0;
int currentPlayer = 1;
and define the following struct game_state:
struct game_state
{
int p1_cur_amt;
int p2_cur_amt;
int p1_cur_pos;
...