0
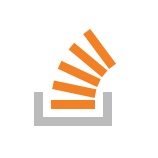
You could initialize the string with the path, you wouldn't even need to know the size (which is returned by GetWindowsDirectoryA as stated in the comment section), but it's advisable to use it given that it does optimize std::string initialization.
Example:
std::string GetOSFolder() {
char ...