0
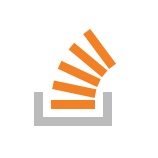
What I want/need to accomplish is to read multipliable numbers within a text file.
I'm still a beginner to programming, and I'm unsure what to do.
Here's what my text file looks like:
23 35 93 55 37 85 99 6 86 2 3 21 30 9 0 89 63 53 86 79
Here's what my code looks like:
FILE* infile = fopen("...