0
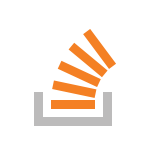
I have a class like this:
class GameEvent:
__metaclass__=GameEventMeta
do_not_register=True
id=None
priority=0
default_label=None
default_args=[]
@classmethod
def process(event,handler_id,*args):
handler=getattr(event,"on_"+handler_id,None)
if callabl...