-1
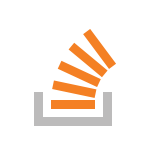
I am not familiar enough with the memory layout of objects that contain virtual bases
Virtual bases (and base class subobjects that have virtual bases) are not in general constructed nor represented like complete objects of the same types, as opposed to any other subobject which has the sam...