1
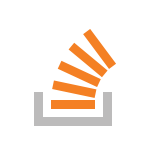
I would recommend you use DataFame(in Spark 2.0 i.e DataSet[Row]) as is, because DataSet uses Encoders so that it will have very little memory footprint than RDD.
val destination = spark.read
.options(options)
.format("jdbc")
.load()
If you want concat columns by delimiter you can...