0
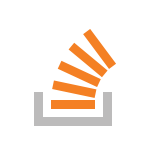
I have some hard to solve crashes. They happen in application from time to time not in regular way. I think the problem maybe with some race conditions and synchronizations.
I am using such pattern.
1) reloadData()
2) reloadSection1(), reloadSection2(), reloadSection2(), etc.
3) I have tap...