-1
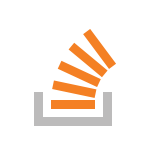
I have written a function in C that pops the first node off of a linked list and makes changes accordingly so the list starts at the next node. However, this results in a segmentation fault and I am not sure why.
I have tried reading through Valgrind documents and reanalyzing my functions but I ...