1
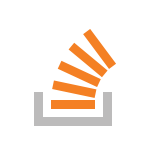
For RawGit, you get content-type: application/javascript;charset=utf-8 as expected, and GitHub gives Content-Type: text/plain; charset=utf-8. That seems to be the only difference.
I checked your example and found that when using RawGit (and getting a script response), the success callback wouldn...