0
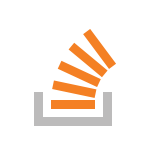
I was making a C program to convert 12 hour clock into 24 hour clock with the format of input being HH:MM:SSAM or HH:MM:SSPM and 24hr clock output being HH:MM:SS
#include <stdio.h>
#include <stdlib.h>
int main(){
char *time = malloc(11 * sizeof(char));
scanf("%11s", time);
if (time[...