2
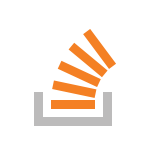
Due to a quirk of the language, Fib() and make_fibonacci_set(), as written, will have infinite recursion (specifically, to my understanding, the problem is that while only one branch is chosen, both are evaluated; this causes the compiler to instantiate the templates required by the recursive bra...