-1
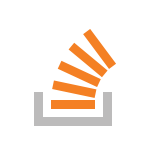
I'm creating a program for fun just to see what you can do with drawings on winforms and I thought of something but have no idea how to do it.
I draw a circle around the cursor as it moves like this:
protected override void OnPaint(PaintEventArgs e)
{
DrawTest(e.Graphics);
base.OnPaint(...