0
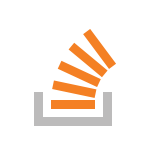
I'm trying to access the edges_ inside my Node Struct, so I can do a for-loop to copy the edges over to a new graph object for my copy constructor.
I'm getting the following error which confuses me when I try to access the edges_ in node.
tests/Graph.tem:280:24: error: ‘struct std::pair<const s...