1
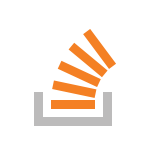
You could use structs instead of dictionaries.
Quick example, let's make a Bus object:
struct Bus {
var name: String
var direction: String
var times: [NSDate]
}
It holds the values for each bus: name of the bus, its direction, and an array of dates for the bus times.
Of course it...